Apollo 9.9 - ADO.NET Data Provider
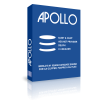
Included with Apollo Embedded 9.9
Overview
The Apollo ADO.NET Data Provider is a fully managed .NET Data Provider that lets .NET developers build WinForm and ASP.NET applications using Visual Studio (Community, Professional and Enterprise editions) and RAD Studio for .NET . Supports C#, VB.NET and other CLR-compliant languages using Visual Studio and Embarcadero, Borland and CodeGear IDEs.
Key Features
- 32-bit and 64-bit support
- Create ASP.NET, WPF and WinForms applications
- Create, update and manage Clipper and FoxPro DBF/Xbase database files from code
- Compatible with .NET data bound controls and 3rd party components
- Easily scalable from embedded to client/server configuration with 5 lines of code
- Samples with full source code
- Royalty free unlimited distribution
Supported .NET Frameworks
- .NET 6.0, 5.0, 4.8, 4.7.2, 4.7.1, 4.7, 4.6.2, 4.6.1, 4.6, 4.5.2, 4.5.1, 4.5, 4.0, 3.5, 3.0, 2.0
Supported .NET IDEs
- Visual Studio Community, Professional and Enterprise
2022, 2019, 2017, 2015, 2013, 2012, 2010, 2008, 2005 - RAD Studio 11 Alexandria, 10.3 Rio, 10.2 Tokyo, 10.1 Berlin, 10 Seattle,
XE8, XE7, XE6, XE5, XE4, XE3, XE2, XE - Delphi 11 Alexandria, 10.3 Rio, 10.2 Tokyo, 10.1 Berlin, 10 Seattle,
XE8, XE7, XE6, XE5, XE4, XE3, XE2, XE - C++Builder 11 Alexandria, 10.3 Rio, 10.2 Tokyo, 10.1 Berlin, 10 Seattle,
XE8, XE7, XE6, XE5, XE4, XE3, XE2, XE
Supported Languages
- All .NET compliant languages
- Samples included in C# and VB.NET
Components
ADO.NET Data Provider
-
ApolloDataAdapter
Works like SqlClient. Fills datasets with data defined by SQL commands and queries from ApolloCommands. -
ApolloCommand
Works much like SqlCommand. Runs SQL-92 queries and commands to manage Clipper and FoxPro 2.6 data. The Query object supports all the common SQL statements such as SELECT, INSERT, UPDATE, DELETE and more. -
ApolloConnection
Works much like SqlConnection. Connects to DBF/Xbase database files and allows you to manage database level settings such as encryption. -
ApolloDataSet
Unique to Apollo. Works much like DataSet. Connects databound controls to DBF/Xbase data tables using high-speed, low overhead DDA objects. More efficient than the standard .NET disconnected datasets yet provides all the convenience of databound controls support, including the DataGrid. -
ApolloDataReader (non-visual)
Works much like SqlDataReader. ApolloTable is modeled after DataSet to provide direct data access with live data cursors to Apollo tables without the overhead of ADO.NET. ApolloTable provides high speed direct table access that is faster than ADO.NET.
Direct Data Access™ Objects
-
ApolloTable (DDA non-visual)
Unique to Apollo. ApolloTable is modeled after Dataset to provide direct data access with live data cursors to Apollo tables without the overhead of ADO.NET. Connects to Apollo tables allowing you to add, delete, update, filter and find data, along with other table related operations. ApolloTable provides high speed direct table access that is faster than ADO.NET. -
ApolloDatabase (DDA non-visual)
Unique to Apolla. ApolloDatabase works with ApolloTable to provide direct data access to DBF/Xbase data files without the overhead of ADO.NET.
Using & Sample Code
Applies to:
- Visual Studio 2022, 2019, 2017, 2015, 2013, 2012, 2010, 2008, 2005 and 2003
- .NET 4.8, 4.7.2, 4.7.1, 4.7, 4.6.2, 4.6.1, 4.6, 4.5.2, 4.5.1, 4.5, 4.0, 3.5, 3.0, 2.0
Installing Apollo into Visual Studio
- Run Visual Studio
- Create a new project or new form solution or use the one made by the Editor Wizard
- Select View Designer mode to see the application form in the editor
- Select the Toolbox icon and select the Data tab on the Toolbox
- Right-click and select "Add/Remove Items..."
-
In the Customize Toolbox dialog, press the Browse
button and select one of the following:
for Visual Studio 2010 (.NET 4.0):
C:\Apollo\[majorversion.minorversion]\NET\Provider\Apollo.Provider.DLL
- Click ok on the hilited items ApolloCommand, ApolloConnection, ApolloDataAdapter.
- VS will hilite and install the Apollo components into the Toolbox dialog
Creating your first .NET application
Apollo .NET Data Provider is a non-visual component (i.e. no wizards). See the included .NET demo for easy-to-follow code. The demo is available for each version of .NET and is located in each .NET version sub-directory as follows:
- C:\Apollo\[majorversion.minorversion]\NET\Samples\Demo\cs
Apollo ADO.NET Data Provider is compatible with the ADO.NET architecture and provides SQL-92 support for disconnected SQL-based data management, exactly like the SqlClient Provider. The Apollo .NET Data Provider components include ApolloConnection, ApolloDataAdapter, ApolloDataReader, ApolloCommand and each work with databound controls and 3rd party products that support ADO.NET.
C# sample using the Apollo Data Provider
// Use the Apollo
Data Provider as you would any other Data Provider
// Create a connection to a Apollo
database
ApolloConnection conn = new
ApolloConnection();
conn.ConnectionString = "Data Source =
C:\\MyData\\MyDB.DBF";
// Set up a DataAdapter and
Dataset for a table
ApolloDataAdapter adapterPerson = new
ApolloDataAdapter();
DataSet dsPerson = new
DataSet();
adapterPerson.SelectCommand = new ApolloCommand("SELECT * FROM
Person", conn);
// Fill the DataAdapter with data
and show it in the
grid
dsPerson.Clear();
adapterPerson.Fill(dsPerson,"Person");
gridPerson.DataSource
= dsPerson;
gridPerson.DataMember = "Person";
DDA is unique to Apollo. DDA consists of 3 .NET objects: ApolloDatabase, ApolloTable and the ApolloDataSet. Together, these objects give you total control over a DBF/Xbase database and provide an intuitive, yet powerful object-oriented method of managing data, sometimes referred to as "live cursors" or "navigational control". DDA lets you navigate dynamically through data tables without having to load the entire table or dataset into memory. This gives DDA a big boost in performance over ADO.NET's disconnected data model. The ApolloDataSet allows DDA to be fully comaptible with all databound controls.
C# sample using the Apollo DDA objects
// Use the Apollo
Direct Data Access objects
// Create a
connection object to a Apollo database
ApolloDatabase vDB = new
ApolloDatabase("C:\\MyData\\MyDB.DBF");
// Create
a table object
ApolloTable vTable = new
ApolloTable(vDB);
vTable.TableName = "Person";
// Define local vars to hold the data
string
fname;
string lname;
boolean married;
integer age;
// Connect to the database and open the
table
vDB.Connect();
vTable.Open();
// Traverse the table to get the records
while(
!vTable.EndOfSet() ){
fname = vTable.GetString(
"FirstName");
lname = vTable.GetString(
"LastName");
married = vTable.GetBoolean(
"Married");
age = vTable.GetInt32(
"Age");
WriteToScreen( fname, lname, married,
age);
// move to the next
record
vTable.Next();
};
// Close our
connections
vTable.Close();
vDB.Close();
Architecture Diagrams
Single User - Local Access
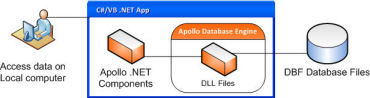
Single User - File Share or LAN Data Access
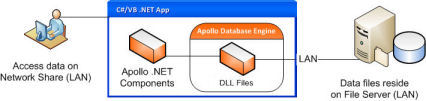
Multiple Users - File Share or LAN Data Access
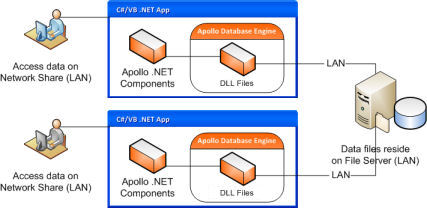
Multiple Users - Web Data Access
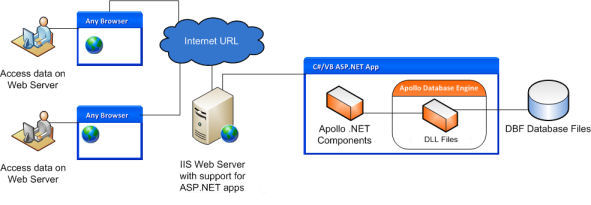
Multiple Users - Client/Server Access
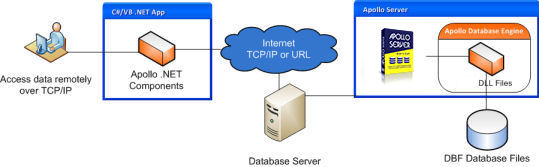
Multiple Users - Client/Server Access with Apollo Relay Server
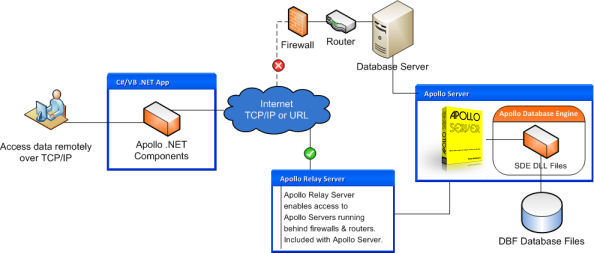
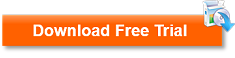
Components
Apollo is an EXCELLENT product which I use for EVERY application I write which requires a database, and nowadays that means all of them.
Apollo just got Hardcore!
I love your products. Applications I developed with
Apollo over the last decade have aced the test of time, with heavy daily use. I purchased the upgrade today.
Congratulations on winning Best Database Engine
Award!
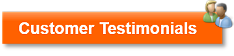